if constexpr
and generic lambdas so that code could use the type if it is defined, while still being accepted by the compiler (and being discarded) if the type is not defined.However, our usage had a few issues, some minor annoyance, some more frustrating.
- You had to say
struct
all the time. - If the type didn’t exist, the act of naming it caused the type to be injected into the current namespace, not the namespace you expected the type to be in.
- You must use the
struct
technique with an unqualified name. You can’t use it to probe a type that you didn’t import into the current namespace.
We can fix all three of the problems with a single solution: Predeclare the type in the desired namespace.
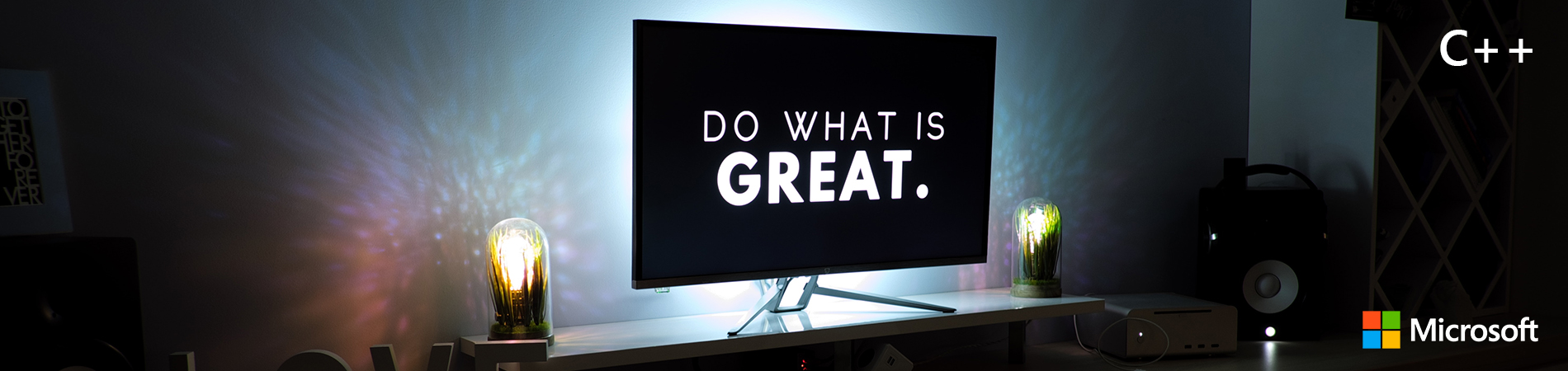