In this article, we will consider the process of setting up a React Native environment using expo-cli, Typescript, and Jest.
Typescript will help us avoid development mistakes and write a more efficient mobile application.
Modern tools allow integrating Typescript into the development environment. We can also use VS Code that supports Typescript.
Integration with React Native will give us the opportunity to use the auto-completion service, code navigation, and refactoring.
Expo is a toolkit that simplifies the creation of native React applications. This tutorial will give you an idea of how you can quickly create native React applications using Expo.
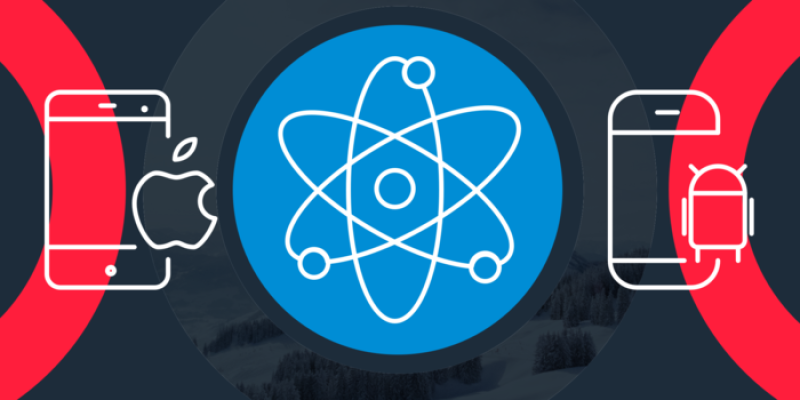
First, we install all the dependencies needed to create the application template from yarn.
yarn global add expo-cli
Next, we initialize the React Native application and select the empty Typescript template.
~/ expo init exampleApp
? Choose a template: expo-template-blank-typescript
Please enter a few initial configuration values.
? Yarn v1.15.2 found. Use Yarn to install dependencies? Yes
Now we can launch the project and begin developing the application.
cd exampleApp
yarn start
Let's configure tslint to use VSCode or another editor correctly and see errors at the development stage. This will ensure a uniform code style and prevent complications.
First we install the tslint package in the global directory:
yarn global add tslint
Next we create the tslint configuration:
tslint --init
This command will create the tslint.json file with the following configuration:
Next, we check the typescript files using our configuration:
tslint 'src/**/*.ts'
After that, VS Code will automatically use the configuration file to validate the code in the editor. Type errors will be displayed in the editor in the process of app development.
Also, for convenience, we add commands for yarn. These commands can be used for local development or for verification at the stage of continuous integration.
Tslint also makes it possible to extend validation rules by installing plugins. At this point, we will add the eslint rules support.
yarn add tslint-eslint-rules --dev
To add a plugin to the tslint configuration, we add the plugin name to the “extends” field:
Jest is a framework for testing the Javascript code. It facilitates the testing, support, and development of React Native applications.
First you need to install the framework and add typescript support, since the codebase was written with support for static typing.
yarn add --dev jest ts-jest @types/jest
It is also worth installing additional packages:
And the last step is to create a configuration file for the tests:
yarn ts-jest config:init
This command will generate a configuration file from the template. We will tailor it to the React Native environment. The file should look like this:
And finally, we should add the command to run the tests in package.json:
«test»: «yarn test»
Let's try to write a sample test for Jest. To do this, we will create an App.test.tsx file:
To run the tests, we execute the previously created yarn command:
If you do all of the above correctly, tests should pass successfully. But you will still not be able to write tests for components. Let's expand the environment for testing React Native components.
We need one more package — react-test-renderer. It provides a special rendering engine for React with a Javascript structure on the output. Therefore, we do not need to configure DOM or native modules in the test environment.
yarn add -D react-test-renderer
Next we update our App.test.tsx file with a simple test for the App.tsx component.
We can test native components in the test environment. In this example, we have obtained an array of child elements for the Text tag. This is a native component from the React Native package.
This technology stack has allowed us to quickly create an environment for developing native applications. Business logic based on static types makes the application more stable. Typescript's strong typing also helps avoid coding errors.
Test development for React Native components within Jest is exactly the same as for regular React applications.
I hope this article will help you overcome the initial stage of setting up an environment for developing React Native applications.
Typescript will help us avoid development mistakes and write a more efficient mobile application.
Modern tools allow integrating Typescript into the development environment. We can also use VS Code that supports Typescript.
Integration with React Native will give us the opportunity to use the auto-completion service, code navigation, and refactoring.
Expo is a toolkit that simplifies the creation of native React applications. This tutorial will give you an idea of how you can quickly create native React applications using Expo.
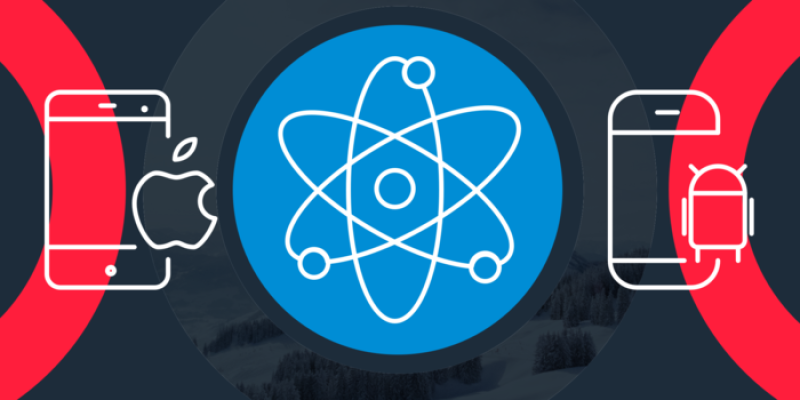
Creating a Project
First, we install all the dependencies needed to create the application template from yarn.
yarn global add expo-cli
Next, we initialize the React Native application and select the empty Typescript template.
~/ expo init exampleApp
? Choose a template: expo-template-blank-typescript
Please enter a few initial configuration values.
? Yarn v1.15.2 found. Use Yarn to install dependencies? Yes
Now we can launch the project and begin developing the application.
cd exampleApp
yarn start
Tslint Configuration
Let's configure tslint to use VSCode or another editor correctly and see errors at the development stage. This will ensure a uniform code style and prevent complications.
First we install the tslint package in the global directory:
yarn global add tslint
Next we create the tslint configuration:
tslint --init
This command will create the tslint.json file with the following configuration:
{
"extends": ["tslint:recommended"],
"jsRules": {},
"rules": {},
"rulesDirectory": []
}
Next, we check the typescript files using our configuration:
tslint 'src/**/*.ts'
After that, VS Code will automatically use the configuration file to validate the code in the editor. Type errors will be displayed in the editor in the process of app development.
Also, for convenience, we add commands for yarn. These commands can be used for local development or for verification at the stage of continuous integration.
"scripts": {
"lint": "tslint '*.ts*'"
}
Tslint also makes it possible to extend validation rules by installing plugins. At this point, we will add the eslint rules support.
yarn add tslint-eslint-rules --dev
To add a plugin to the tslint configuration, we add the plugin name to the “extends” field:
"extends": [
"tslint:recommended",
"tslint-eslint-rules"
]
Jest and tests on Typescript
Jest is a framework for testing the Javascript code. It facilitates the testing, support, and development of React Native applications.
First you need to install the framework and add typescript support, since the codebase was written with support for static typing.
yarn add --dev jest ts-jest @types/jest
It is also worth installing additional packages:
- ts-jest — for compiling and processing typescript test code in Javascript
- @ types / jest — for adding types from the Jest environment
- react-native-testing-library — for rendering React components without DOM
And the last step is to create a configuration file for the tests:
yarn ts-jest config:init
This command will generate a configuration file from the template. We will tailor it to the React Native environment. The file should look like this:
module.exports = {
jest: {
preset: "react-native",
transform: {
"^.+\\.js$": "./node_modules/react-native/jest/preprocessor.js",
"\\.(ts|tsx)$": "ts-jest"
},
globals: {
"ts-jest": {
tsConfig: "tsconfig.json"
}
},
moduleFileExtensions: ["ts", "tsx", "js"],
testRegex: "(/__tests__/.*|\\.(test|spec))\\.(ts|tsx|js)$"
}
};
And finally, we should add the command to run the tests in package.json:
«test»: «yarn test»
Writing Tests for Jest
Let's try to write a sample test for Jest. To do this, we will create an App.test.tsx file:
export const helloFn = (name?: String = "World") => `Hello, ${$name}`;
describe("hello function", () => {
it("should return `hello world`", () => {
expect(helloFn()).toEqual(`Hello, World`);
});
it("should return `hello name`", () => {
expect(helloFn("Zuck")).toEqual(`Hello, Zuck`);
});
});
To run the tests, we execute the previously created yarn command:
yarn test
PASS ./App.test.tsx
hello function
✓ should return `hello world` (4ms)
✓ should return `hello name` (1ms)
Test Suites: 1 passed, 1 total
Tests: 2 passed, 2 total
Snapshots: 0 total
Time: 1.316s
Ran all test suites.
Done in 2.74s.
If you do all of the above correctly, tests should pass successfully. But you will still not be able to write tests for components. Let's expand the environment for testing React Native components.
We need one more package — react-test-renderer. It provides a special rendering engine for React with a Javascript structure on the output. Therefore, we do not need to configure DOM or native modules in the test environment.
yarn add -D react-test-renderer
Next we update our App.test.tsx file with a simple test for the App.tsx component.
import React from "react";
import renderer from "react-test-renderer";
import App from "./App";
describe("App", () => {
it("should display welcome message", () => {
const tree = renderer.create(<App />);
expect(tree.toJSON()).toMatchSnapshot();
expect(tree.root.findByType("Text").children).toContain(
"Open up App.tsx to start working on your app!"
);
});
});
We can test native components in the test environment. In this example, we have obtained an array of child elements for the Text tag. This is a native component from the React Native package.
Conclusion
This technology stack has allowed us to quickly create an environment for developing native applications. Business logic based on static types makes the application more stable. Typescript's strong typing also helps avoid coding errors.
Test development for React Native components within Jest is exactly the same as for regular React applications.
I hope this article will help you overcome the initial stage of setting up an environment for developing React Native applications.